How to Make a Telegram Bot: A Complete Dev Guide
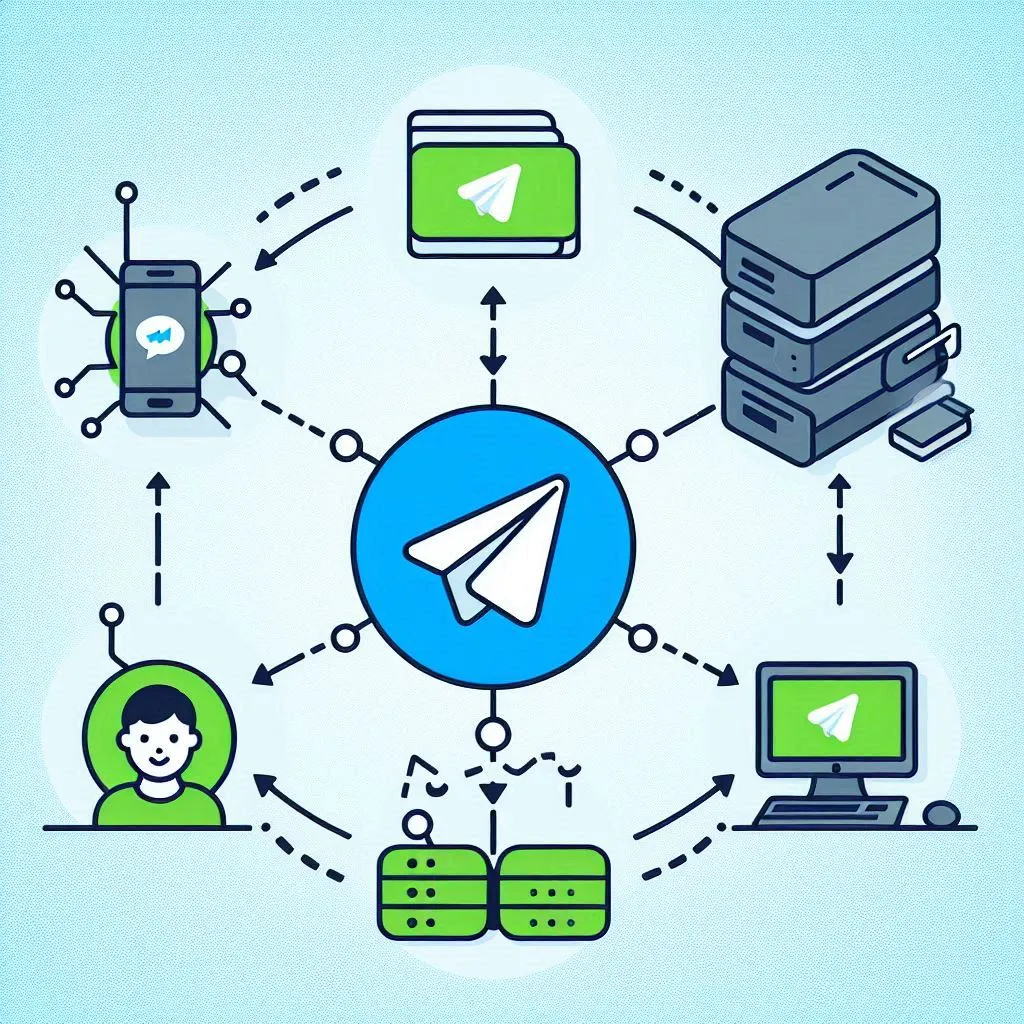
Telegram bots are powerful tools that can automate tasks, provide services, and enhance user experiences within the Telegram messaging platform. In this detailed guide, we’ll walk you through the process of creating your own Telegram bot using various programming languages, with a focus on Go (Golang), Python, PHP, JavaScript, and Ruby.
Understanding Telegram Bots
Telegram bots are special accounts that don’t require a phone number to set up. They can perform a wide range of functions, from sending notifications to integrating with other services.
Key Features of Telegram Bots:
- Can handle messages and commands
- Support inline queries
- Can be integrated with external services
- Can send various types of media
Setting Up Your Development Environment
Before we start coding our bot, let’s set up our development environment.
1. Choose Your Programming Language
We’ll provide examples in Go, Python, PHP, JavaScript, and Ruby. Choose the language you’re most comfortable with.
2. Install Dependencies
Depending on your chosen language, install the necessary dependencies:
- Go:
go get -u github.com/bakatz/telegram-bot-api
- Python:
pip install python-telegram-bot
- PHP:
composer require irazasyed/telegram-bot-sdk
- JavaScript:
npm install node-telegram-bot-api
- Ruby:
gem install telegram-bot-ruby
3. Choose an IDE
We recommend using Visual Studio Code with the appropriate language extensions for the best development experience.
Creating Your Telegram Bot
Now that we have our environment set up, let’s create our bot.
1. Get a Bot Token
- Open Telegram and search for the BotFather.
- Start a chat and send the command
/newbot
. - Follow the prompts to choose a name and username for your bot.
- Save the API token provided by BotFather.
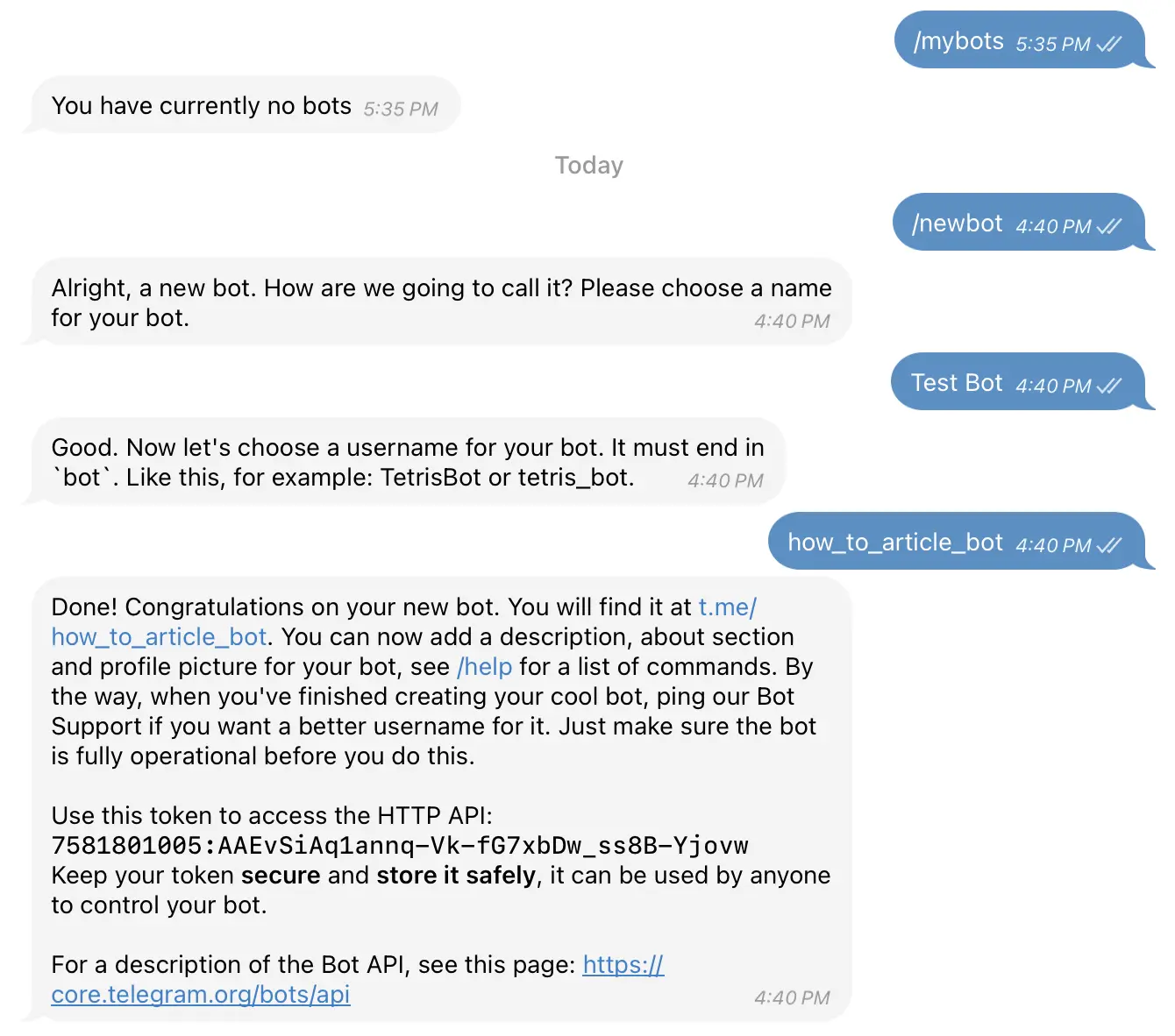
2. Write the Bot Code
Here are examples of a basic echo bot in different languages:
Go (using github.com/bakatz/telegram-bot-api)
package main
import (
"log"
"os"
tgbotapi "github.com/bakatz/telegram-bot-api/v5"
)
func main() {
bot, err := tgbotapi.NewBotAPI(os.Getenv("TELEGRAM_APITOKEN"))
if err != nil {
log.Panic(err)
}
bot.Debug = true
log.Printf("Authorized on account %s", bot.Self.UserName)
u := tgbotapi.NewUpdate(0)
u.Timeout = 60
updates := bot.GetUpdatesChan(u)
for update := range updates {
if update.Message == nil {
continue
}
log.Printf("[%s] %s", update.Message.From.UserName, update.Message.Text)
msg := tgbotapi.NewMessage(update.Message.Chat.ID, update.Message.Text)
msg.ReplyToMessageID = update.Message.MessageID
bot.Send(msg)
}
}
Python
import os
from telegram.ext import Updater, CommandHandler, MessageHandler, Filters
def echo(update, context):
context.bot.send_message(chat_id=update.effective_chat.id, text=update.message.text)
def main():
updater = Updater(token=os.environ['TELEGRAM_APITOKEN'], use_context=True)
dispatcher = updater.dispatcher
echo_handler = MessageHandler(Filters.text & ~Filters.command, echo)
dispatcher.add_handler(echo_handler)
updater.start_polling()
updater.idle()
if __name__ == '__main__':
main()
PHP
<?php
require_once __DIR__ . '/vendor/autoload.php';
use Telegram\Bot\Api;
$telegram = new Api(getenv('TELEGRAM_APITOKEN'));
$response = $telegram->getUpdates();
foreach ($response as $update) {
$message = $update->getMessage();
$chatId = $message->getChat()->getId();
$text = $message->getText();
$telegram->sendMessage([
'chat_id' => $chatId,
'text' => $text
]);
}
JavaScript (Node.js)
const TelegramBot = require('node-telegram-bot-api');
const token = process.env.TELEGRAM_APITOKEN;
const bot = new TelegramBot(token, {polling: true});
bot.on('message', (msg) => {
const chatId = msg.chat.id;
bot.sendMessage(chatId, msg.text);
});
Ruby
require 'telegram/bot'
token = ENV['TELEGRAM_APITOKEN']
Telegram::Bot::Client.run(token) do |bot|
bot.listen do |message|
bot.api.send_message(chat_id: message.chat.id, text: message.text)
end
end
3. Run Your Bot
Set your bot token as an environment variable and run the bot using the appropriate command for your chosen language.
Adding Features to Your Bot
Now that we have a basic bot running, let’s add some features, specifically focusing on handling commands. We’ll provide examples in all the languages we’ve covered.
Handling Commands
Here are examples of how to handle commands in different languages:
Go (using github.com/bakatz/telegram-bot-api)
package main
import (
"log"
"os"
tgbotapi "github.com/bakatz/telegram-bot-api"
)
func main() {
bot, err := tgbotapi.NewBotAPI(os.Getenv("TELEGRAM_APITOKEN"))
if err != nil {
log.Panic(err)
}
bot.Debug = true
log.Printf("Authorized on account %s", bot.Self.UserName)
u := tgbotapi.NewUpdate(0)
u.Timeout = 60
updates := bot.GetUpdatesChan(u)
for update := range updates {
if update.Message == nil {
continue
}
if update.Message.IsCommand() {
msg := tgbotapi.NewMessage(update.Message.Chat.ID, "")
switch update.Message.Command() {
case "start":
msg.Text = "Welcome! I'm your new Telegram bot."
case "help":
msg.Text = "I can help you with various tasks. Use /commands to see what I can do."
case "commands":
msg.Text = "Available commands:\n/start - Start the bot\n/help - Get help\n/commands - Show available commands"
default:
msg.Text = "Unknown command. Use /help to see available commands."
}
bot.Send(msg)
} else {
// Echo the message (as before)
msg := tgbotapi.NewMessage(update.Message.Chat.ID, update.Message.Text)
msg.ReplyToMessageID = update.Message.MessageID
bot.Send(msg)
}
}
}
Python
import os
from telegram import Update
from telegram.ext import Updater, CommandHandler, MessageHandler, Filters, CallbackContext
def start(update: Update, context: CallbackContext) -> None:
update.message.reply_text('Welcome! I\'m your new Telegram bot.')
def help_command(update: Update, context: CallbackContext) -> None:
update.message.reply_text('I can help you with various tasks. Use /commands to see what I can do.')
def commands(update: Update, context: CallbackContext) -> None:
update.message.reply_text('Available commands:\n/start - Start the bot\n/help - Get help\n/commands - Show available commands')
def echo(update: Update, context: CallbackContext) -> None:
update.message.reply_text(update.message.text)
def main() -> None:
updater = Updater(token=os.environ['TELEGRAM_APITOKEN'])
dispatcher = updater.dispatcher
dispatcher.add_handler(CommandHandler("start", start))
dispatcher.add_handler(CommandHandler("help", help_command))
dispatcher.add_handler(CommandHandler("commands", commands))
dispatcher.add_handler(MessageHandler(Filters.text & ~Filters.command, echo))
updater.start_polling()
updater.idle()
if __name__ == '__main__':
main()
PHP
<?php
require_once __DIR__ . '/vendor/autoload.php';
use Telegram\Bot\Api;
$telegram = new Api(getenv('TELEGRAM_APITOKEN'));
$telegram->commandsHandler(true);
$telegram->addCommand(Telegram\Bot\Commands\HelpCommand::class);
$telegram->addCommand(
Telegram\Bot\Commands\Command::class,
'start',
'Welcome! I\'m your new Telegram bot.'
);
$telegram->addCommand(
Telegram\Bot\Commands\Command::class,
'commands',
"Available commands:\n/start - Start the bot\n/help - Get help\n/commands - Show available commands"
);
$response = $telegram->getUpdates();
foreach ($response as $update) {
$message = $update->getMessage();
$chatId = $message->getChat()->getId();
$text = $message->getText();
if ($message->isCommand()) {
$telegram->triggerCommand($text, $update);
} else {
$telegram->sendMessage([
'chat_id' => $chatId,
'text' => $text
]);
}
}
JavaScript (Node.js)
const TelegramBot = require('node-telegram-bot-api');
const token = process.env.TELEGRAM_APITOKEN;
const bot = new TelegramBot(token, {polling: true});
bot.onText(/\/start/, (msg) => {
const chatId = msg.chat.id;
bot.sendMessage(chatId, 'Welcome! I\'m your new Telegram bot.');
});
bot.onText(/\/help/, (msg) => {
const chatId = msg.chat.id;
bot.sendMessage(chatId, 'I can help you with various tasks. Use /commands to see what I can do.');
});
bot.onText(/\/commands/, (msg) => {
const chatId = msg.chat.id;
bot.sendMessage(chatId, 'Available commands:\n/start - Start the bot\n/help - Get help\n/commands - Show available commands');
});
bot.on('message', (msg) => {
const chatId = msg.chat.id;
if (!msg.text.startsWith('/')) {
bot.sendMessage(chatId, msg.text);
}
});
Ruby
require 'telegram/bot'
token = ENV['TELEGRAM_APITOKEN']
Telegram::Bot::Client.run(token) do |bot|
bot.listen do |message|
case message.text
when '/start'
bot.api.send_message(chat_id: message.chat.id, text: "Welcome! I'm your new Telegram bot.")
when '/help'
bot.api.send_message(chat_id: message.chat.id, text: "I can help you with various tasks. Use /commands to see what I can do.")
when '/commands'
bot.api.send_message(chat_id: message.chat.id, text: "Available commands:\n/start - Start the bot\n/help - Get help\n/commands - Show available commands")
else
bot.api.send_message(chat_id: message.chat.id, text: message.text)
end
end
end
These examples demonstrate how to handle basic commands (/start, /help, /commands) and echo back non-command messages in each language. You can extend these examples to add more complex functionality to your bot.
Deploying Your Bot
To keep your bot running 24/7, you’ll need to deploy it to a server.
1. Choose a Hosting Provider
Popular hosting platforms offer a variety of services, pricing, and ease of use. Here’s a brief overview of the most popular ones:
-
Heroku:
- Ideal for small projects and fast deployments. It abstracts away much of the server management, making it an excellent choice for beginners.
- Setup: Heroku offers an entry-level tier for $5/month that lets you easily deploy apps through Git by pushing to a remote repository. It also provides an easy-to-use web interface for managing your app.
- Features: Heroku handles provisioning, scaling, and monitoring. Add-ons like Redis or PostgreSQL can be installed with one click.
- Usage: Ideal for rapid development and prototyping but may become expensive as your app scales.
-
DigitalOcean:
- A flexible and more customizable solution. You’ll need to manage your own servers (called Droplets) but can choose from a variety of server configurations.
- Setup: DigitalOcean provides one-click apps for popular frameworks or you can deploy your app via a pre-configured VPS. It requires some knowledge of Linux/Unix for setup and management.
- Features: Offers low-cost virtual servers with SSD storage, and has a flexible pricing model. You’ll be responsible for provisioning databases, scaling, and backups. Great for users who need more control over their infrastructure.
- Usage: Good for growing projects where control, scalability, and performance are important.
-
Google Cloud Platform (GCP):
- Enterprise-level infrastructure with a vast array of services (compute, storage, databases). You can scale globally and run your application on the same infrastructure Google uses.
- Setup: GCP is more complex to set up initially but allows for fine-tuned control over your environment. Services like App Engine, Kubernetes Engine, and Cloud Functions provide flexibility for different types of applications.
- Features: Auto-scaling, regional redundancy, and integration with a wide array of services. It’s well-suited for larger applications or those needing complex infrastructure (such as machine learning, big data, etc.).
- Usage: Recommended for large projects, or apps that expect significant growth and need enterprise-grade features.
2. Set Up Version Control
Using version control like Git is crucial for managing code, especially for deployment purposes:
- Git Setup:
- Install Git on your local development environment and initialize a Git repository with
git init
. - Make sure to commit your code frequently with meaningful commit messages. This allows you to track changes, roll back mistakes, and collaborate with others.
- Install Git on your local development environment and initialize a Git repository with
- Connecting to Hosting Providers:
- For platforms like Heroku, you can directly deploy your app by adding the Heroku Git remote:
heroku git:remote -a your-app-name git push heroku master
- For services like DigitalOcean, you can deploy through GitHub Actions or manually using SSH and Git.
- Most cloud platforms have a CI/CD pipeline (e.g., Google Cloud’s Cloud Build) where you can configure automated deployments directly from your repository (GitHub, GitLab, etc.).
- For platforms like Heroku, you can directly deploy your app by adding the Heroku Git remote:
3. Configure Environment Variables
Environment variables allow you to keep sensitive information (e.g., API keys, database URIs) outside of your codebase. Most hosting providers offer simple ways to manage environment variables.
-
Heroku:
- You can set environment variables through the Heroku dashboard or via the Heroku CLI:
heroku config:set BOT_TOKEN=your-bot-token
- These variables will be available in your app at runtime, typically accessed in your code as `process.env.BOT_TOKEN` (for Node.js).
- You can set environment variables through the Heroku dashboard or via the Heroku CLI:
-
DigitalOcean:
- If you’re using a VPS, you can configure environment variables directly in the shell or in your deployment scripts (`export BOT_TOKEN=your-bot-token`), or via Docker Compose if you’re containerizing your app.
-
Google Cloud Platform:
- GCP allows you to set environment variables via the App Engine configuration file (`app.yaml`):
env_variables: BOT_TOKEN: "your-bot-token"
- For other services like Kubernetes, you can use ConfigMaps and Secrets to manage environment variables.
- GCP allows you to set environment variables via the App Engine configuration file (`app.yaml`):
4. Deploy Your Code
Each hosting provider has its own deployment process. Here’s a basic rundown for each:
-
Heroku:
- Once your Git repository is linked to Heroku, you can deploy by simply pushing your code to the Heroku Git remote:
git push heroku master
- Heroku automatically detects the type of app (e.g., Node.js, Python) and sets up the environment accordingly. After the push, your app will build and deploy. You can view the logs to monitor the progress.
- Once your Git repository is linked to Heroku, you can deploy by simply pushing your code to the Heroku Git remote:
-
DigitalOcean:
- Deployments to DigitalOcean depend on how you’ve set up your server. If using a simple VPS, you can SSH into the server and pull your latest code from your repository, or set up a deployment pipeline using GitHub Actions, GitLab CI, or any other CI/CD service.
- If using Docker, you can push your Docker images to a registry (like Docker Hub or GitHub Packages) and then pull and run them on your Droplet. Docker Compose is often used for multi-container setups.
-
Google Cloud Platform:
- If using Google App Engine, you can deploy by running the following command from your terminal:
gcloud app deploy
- For Kubernetes, you would use
kubectl apply
to deploy your manifests, or use Google Kubernetes Engine’s (GKE) integration with CI/CD pipelines. - For Compute Engine, deploy through SSH, Docker, or other orchestration tools like Ansible.
- If using Google App Engine, you can deploy by running the following command from your terminal:
Each platform has robust documentation to help guide you through deployment, including error troubleshooting and scaling options. It’s also a good idea to automate your deployment process with CI/CD tools like GitHub Actions, GitLab CI, or CircleCI.
Best Practices for Telegram Bot Development
- Security: Never hardcode your bot token in your source code.
- Error Handling: Implement robust error handling to prevent crashes.
- Rate Limiting: Respect Telegram’s rate limits to avoid being blocked.
- User Experience: Design clear and intuitive command structures.
- Privacy: Only collect and store necessary user data, and be transparent about it.
Enhancing Your Bot with AI-Powered Moderation
As your Telegram bot gains popularity, managing user interactions becomes increasingly important. This is where AI-powered moderation tools like Watchdog come into play. Watchdog is an AI content moderator specifically designed for Telegram, offering advanced features to keep your users’ conversations safe and on-topic.
Benefits of Using Watchdog:
-
Automated Content Moderation: Watchdog uses advanced AI algorithms to automatically detect and filter out inappropriate content, spam, and offensive language.
-
Customizable Rules: Tailor the moderation rules to fit your bot’s specific needs and community guidelines.
-
Real-time Monitoring: Get instant alerts and take immediate action on potential issues in your bot’s conversations.
-
Multi-language Support: Effectively moderate conversations in multiple languages, expanding your bot’s global reach.
-
Integration with Existing Bots: Easily integrate Watchdog alongside your current Telegram bot setup for seamless moderation.
By incorporating Watchdog into your Telegram bot, you can focus on developing innovative features while ensuring a safe and enjoyable experience for your users. This AI-powered moderation can significantly reduce the manual effort required to manage large-scale bot interactions, allowing your bot to scale efficiently.
Conclusion
Creating a Telegram bot can be an exciting and rewarding project. With this guide, you’ve learned the basics of setting up, coding, and deploying your own bot using various programming languages. As you continue to develop your bot, remember to explore the Telegram Bot API documentation for more advanced features and possibilities.
To ensure the long-term success and scalability of your bot, consider implementing AI-powered moderation tools like Watchdog. These tools can help maintain a positive user experience, protect your community, and allow you to focus on creating innovative features for your bot.
Happy coding, and may your bot bring value and joy to its users!